WalletConnect Modal to Reown AppKit Core
WalletConnect Modal will soon be deprecated. Consequently, projects and developers need to upgrade to Reown AppKit Core. The most basic version of Reown AppKit will contain the traditional WalletConnect Modal with a QR code that users can scan and connect their wallets to.
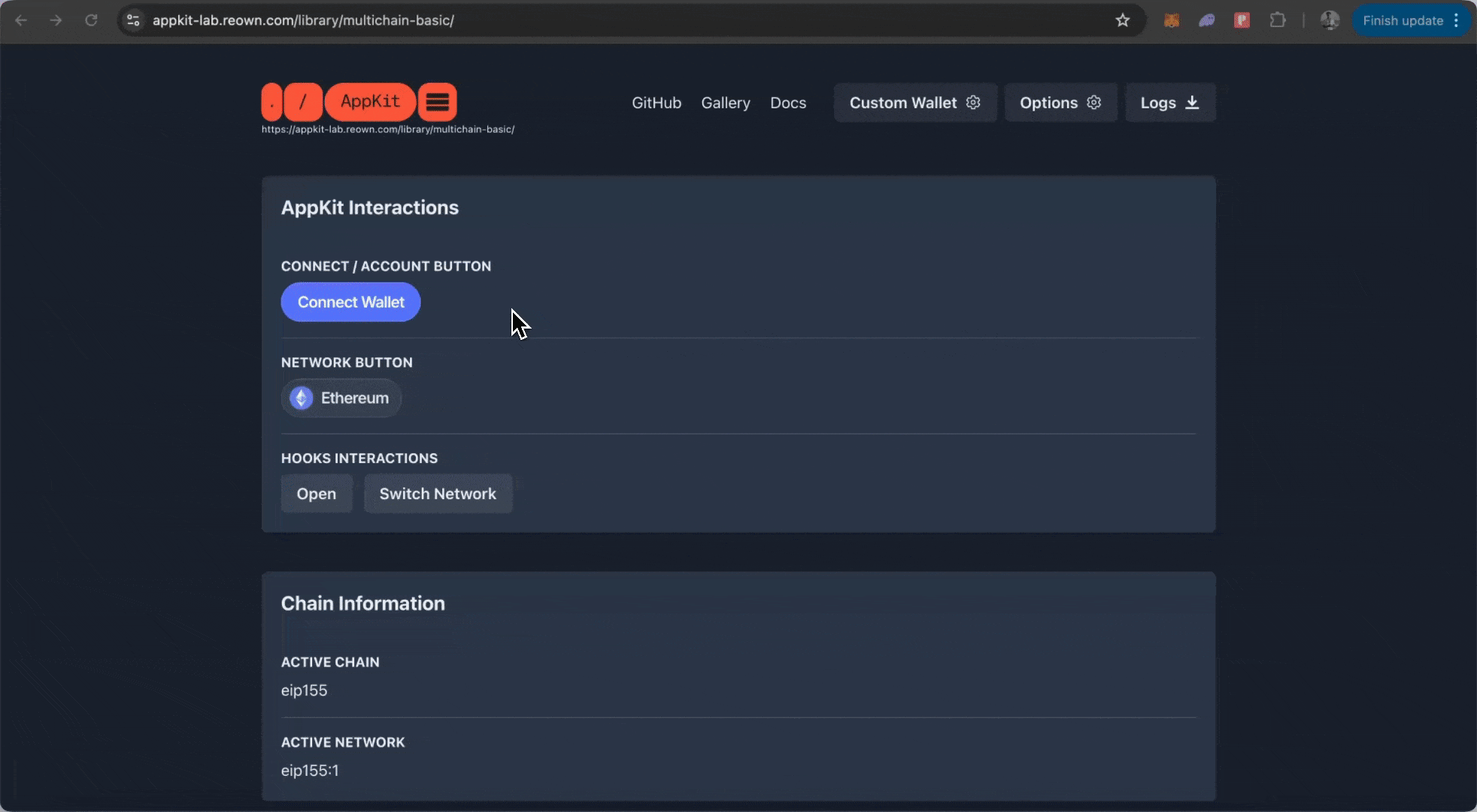
This guide will show you how to upgrade to the most basic version of Reown AppKit. There are three different categories that this guide will be addressing, they are:
Installation
You first need to install the AppKit package in order to get started. You can do this by running the command below.
- npm
- Yarn
- Bun
- pnpm
npm install @reown/appkit
yarn add @reown/appkit
bun add @reown/appkit
pnpm add @reown/appkit
AppKit Basic
This is the easiest way for developers to go multi-chain. AppKit Basic utilizes the UniversalProvider
underneath and is a ready-to-use solution with hooks and methods.
- React
- Vue
- JavaScript
import { createAppKit } from '@reown/appkit/react'
import { mainnet } from '@reown/appkit/networks'
const modal = createAppKit({
adapters: [], //pass an empty array to only use WalletConnect QR
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
networks: [mainnet]
})
import { createAppKit } from '@reown/appkit'
import { mainnet } from '@reown/appkit/networks'
const modal = createAppKit({
adapters: [], //pass an empty array to only use WalletConnect QR
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
networks: [mainnet]
})
import { createAppKit } from '@reown/appkit/vue'
import { mainnet } from '@reown/appkit/networks'
const modal = createAppKit({
adapters: [], //pass an empty array to only use WalletConnect QR
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
networks: [mainnet]
})
You can also refer to the "Multichain" section under AppKit "Core" for installation. Click here to learn more.
Examples
Below are the examples for the corresponding library/programming language.
Ethereum Provider
The Ethereum Provider implementation remains the same as before. Below is an example of how you can configure it:
import { EthereumProvider } from '@walletconnect/ethereum-provider'
const provider = await EthereumProvider.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
showQrModal: true,
optionalChains: [1, 137, 2020],
/*Optional - Add custom RPCs for each supported chain*/
rpcMap: {
1: 'mainnet.rpc...',
137: 'polygon.rpc...'
}
})
// Connect EthereumProvider, this will open modal
await provider.connect()
Changes Required
Projects and developers don’t need to change anything in their configuration - upgrading the Ethereum Provider is sufficient. Projects will automatically receive the new QR modal UI.
Not all themeVariables
will be compatible with the new UI, as AppKit
uses a different design system than walletConnectModal
Examples
Below are the examples for the corresponding library/programming language.
UniversalProvider with AppKit
Here's how you can configure AppKit with UniversalProvider
.
// NEW IMPLEMENTATION
import { UniversalProvider } from '@walletconnect/universal-provider'
import { createAppKit } from '@reown/appkit'
const provider = await UniversalProvider.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
})
const modal = createAppKit({
projectId: 'YOUR_PROJECT_ID',
networks: [mainnet, solana]
})
// listen to display_uri event and feed modal with uri
provider.on('display_uri', (uri: string) => {
modal.open({ uri, view: 'ConnectingWalletConnectBasic' })
})
// Connect provider, this will trigger display_uri event
await provider.connect({
optionalNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
},
solana: {
methods: ['solana_signMessage', 'solana_signTransaction'],
chains: ['solana:mainnet'],
events: ['chainChanged', 'accountsChanged']
}
}
})
// OLD IMPLEMENTATION
import { UniversalProvider } from '@walletconnect/universal-provider'
import { WalletConnectModal } from '@walletconnect/modal'
const provider = await UniversalProvider.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
})
const modal = new WalletConnectModal({
projectId: 'YOUR_PROJECT_ID',
chains: ['eip155:1', 'solana:mainnet']
})
// listen to display_uri event and feed modal with uri
provider.on('display_uri', (uri: string) => {
modal.openModal({ uri })
})
// Connect provider, this will trigger display_uri event
await provider.connect({
optionalNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
},
solana: {
methods: ['solana_signMessage', 'solana_signTransaction'],
chains: ['solana:mainnet'],
events: ['chainChanged', 'accountsChanged']
}
}
})
Changes
For projects that are currently using the UniversalProvider
with WalletConnectModal
there are two options for you to upgrade.
Option 1
Upgrade to AppKit Basic and pass in the UniversalProvider
.
const provider = await UniversalProvider.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
})
const modal = createAppKit({
projectId: 'YOUR_PROJECT_ID',
networks: [mainnet, solana]
universalProvider: provider
})
Option 2
Replace WalletConnectModal
with AppKit
.
//Old Code
const modal = new WalletConnectModal({
projectId: 'YOUR_PROJECT_ID',
chains: ['eip155:1', 'solana:mainnet']
})
provider.on('display_uri', (uri: string) => {
modal.openModal({ uri })
})
//New Code
const modal = createAppKit({
projectId: 'YOUR_PROJECT_ID',
networks: [mainnet, solana]
})
provider.on('display_uri', (uri: string) => {
modal.open({ uri, view: 'ConnectingWalletConnectBasic' })
})
Examples
Below are the examples for the corresponding library/programming language.
Sign Client with AppKit
Below is how you can use SignClient
with AppKit**.
// Old Implementation
import { SignClient } from '@walletconnect/sign-client'
import { WalletConnectModal } from '@walletconnect/modal'
const signClient = await SignClient.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
})
const modal = new WalletConnectModal({
projectId: 'YOUR_PROJECT_ID',
chains: ['eip155:1']
})
// connect signClient and feed uri to modal
const { uri, approval } = await signClient.connect({
requiredNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
})
if (uri) {
modal.openModal({ uri })
const session = await approval()
modal.closeModal()
}
// New Implementation
import { SignClient } from '@walletconnect/sign-client'
import { createAppKit } from '@reown/appkit'
const signClient = await SignClient.init({
projectId: 'YOUR_PROJECT_ID',
metadata: {
name: 'My Website',
description: 'My Website Description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.githubusercontent.com/u/37784886']
},
})
const modal = createAppKit({
projectId: 'YOUR_PROJECT_ID',
networks: [mainnet]
})
// connect signClient and feed uri to modal
const { uri, approval } = await signClient.connect({
requiredNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
})
if (uri) {
modal.open({ uri, view: 'ConnectingWalletConnectBasic' })
const session = await approval()
modal.close()
}
Changes
For projects and developers that are currently using the SignClient
with WalletConnectModal
there is one option for you to upgrade.
Option 1
Replace WalletConnectModal
with AppKit. Below is what the implementation would look like.
// Old Code
const modal = new WalletConnectModal({
projectId: 'YOUR_PROJECT_ID',
chains: ['eip155:1', 'solana:mainnet']
})
const { uri, approval } = await signClient.connect({
requiredNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
})
if (uri) {
modal.openModal({ uri })
const session = await approval()
modal.closeModal()
}
// New Code
const modal = createAppKit({
projectId: 'YOUR_PROJECT_ID',
networks: [mainnet]
})
// connect signClient and feed uri to modal
const { uri, approval } = await signClient.connect({
requiredNamespaces: {
eip155: {
methods: [
'eth_sendTransaction',
'eth_signTransaction',
'eth_sign',
'personal_sign',
'eth_signTypedData'
],
chains: ['eip155:1'],
events: ['chainChanged', 'accountsChanged']
}
}
})
if (uri) {
modal.open({ uri, view: 'ConnectingWalletConnectBasic' })
const session = await approval()
modal.close()
}
Examples
Below are the examples for the corresponding library/programming language.